Learn practical skills, build real-world projects, and advance your career
Created a year ago
PyTorch Essential Training
with Jonathan Fernandes (LinkedIn Learning)
⇨ Part One: Working with the FMNIST dataset
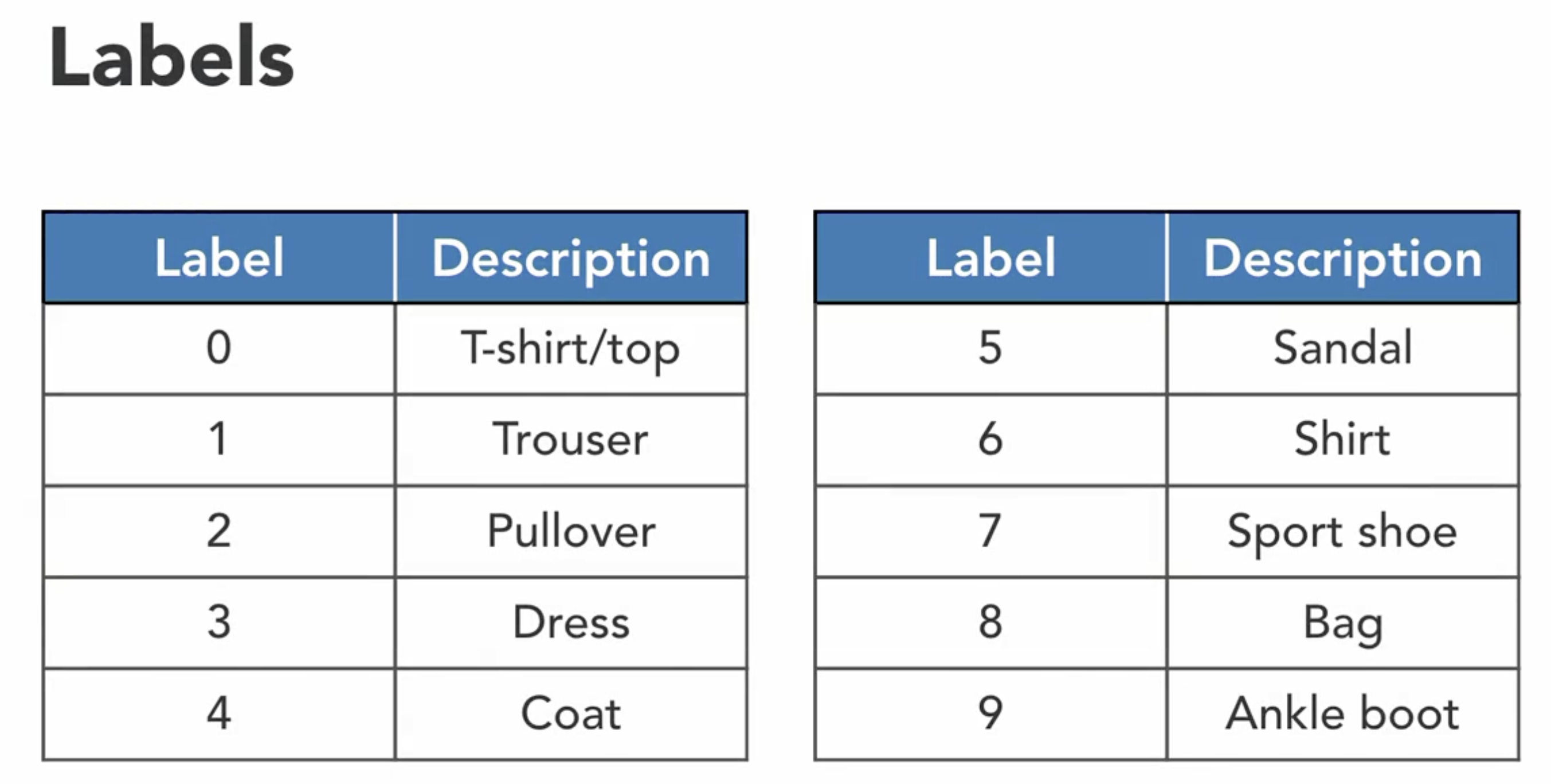
# Setting seeds to try and ensure we have the same results - this is not guaranteed across PyTorch releases.
import torch
torch.manual_seed(0)
torch.backends.cudnn.deterministic = True
torch.backends.cudnn.benchmark = False
from torchvision import datasets, transforms
import torch.nn.functional as F
from torch import nn
from torch import optim
import numpy as np
np.random.seed(0)
%%capture
mean, std = (0.5,), (0.5,)
# Create a transform and normalise data
transform = transforms.Compose([transforms.ToTensor(),
transforms.Normalize(mean, std)
])
# Download FMNIST training dataset and load training data
trainset = datasets.FashionMNIST('~/.pytorch/FMNIST/', download=True, train=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=64, shuffle=True)
# Download FMNIST test dataset and load test data
testset = datasets.FashionMNIST('~/.pytorch/FMNIST/', download=True, train=False, transform=transform)
testloader = torch.utils.data.DataLoader(testset, batch_size=64, shuffle=False)